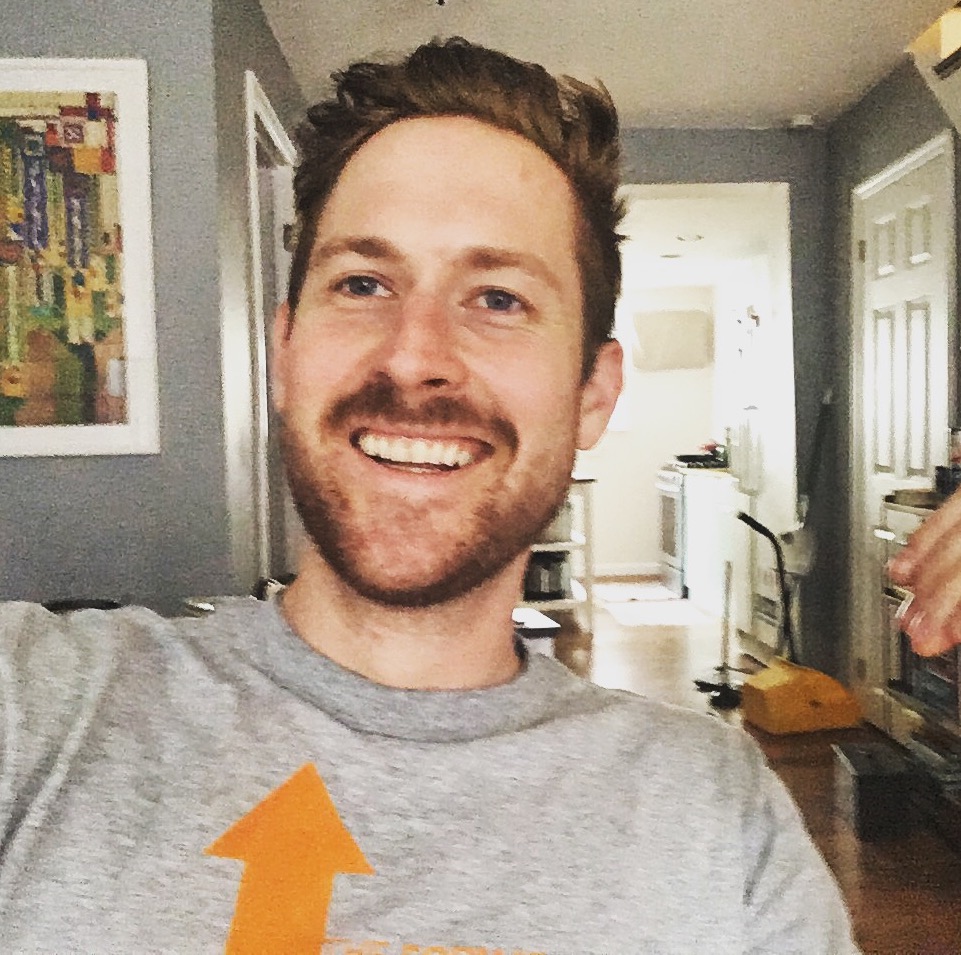
I found some old JS code
I’ve been cleaning out my physical and digital worlds and came upon some of the
earliest JavaScript code I ever wrote. It was a pretty simple slide show I made
to display some of my super hip and artsy black and white analog photos I had
ironically scanned to a digital format for mass consumption. I think I had it
up on GoDaddy via ftp
with just a few hard coded html pages at the time.
Here’s what it looked like when I found it (and it still worked!):
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01//EN">
<html>
<head>
<script language="JavaScript">
var interval = 5000;
var random_display = 1;
var imageDir = "images/";
var imageNum = 0;
imageArray = new Array();
imageArray[imageNum++] = new imageItem( imageDir + "01.jpg");
imageArray[imageNum++] = new imageItem( imageDir + "02.jpg");
imageArray[imageNum++] = new imageItem( imageDir + "03.jpg");
imageArray[imageNum++] = new imageItem( imageDir + "04.jpg");
var totalImages = imageArray.length;
function imageItem(image_location) {
this.image_item = new Image();
this.image_item.src = image_location;
}
function get_ImageItemLocation( imageObj ) {
return( imageObj.image_item.src );
}
function randNum(x, y) {
var range = y - x + 1;
return Math.floor( Math.random() * range ) + x;
}
function getNextImage() {
if (random_display) {
imageNum = randNum(0, totalImages - 1)
}
else{
imageNum = (imageNum + 1) % totalImages;
}
var new_image = get_ImageItemLocation( imageArray[imageNum]);
return(new_image);
}
function getPrevImage() {
imageNum = (imageNum - 1) % totalImages;
var new_image = get_ImageItemLocation( imageArray[imageNum]);
return(new_image);
}
function prevImage(place) {
var new_image = getPrevImage();
document [place].src = new_image;
}
function switchImage(place) {
var new_image = getNextImage();
document[place].src = new_image;
var recur_call = "switchImage('"+place+"')";
timerID = setTimeout(recur_call, interval);
}
</script>
</head>
<!-- <body> -->
<body onLoad="switchImage('slideImg')">
<!-- Slide Show -->
<img name="slideImg" src="images/03.jpg">
<br>
<a href="#" onClick="switchImage('slideImg')">play slide show</a>
<a href="#" onClick="clearTimeout(timerID)"> pause</a>
<a href="#" onClick="prevImage('slideImg'); clearTimeout(timerID)"> previous</a>
<a href="#" onClick="switchImage('slideImg'); clearTimeout(timerID)">next </a>
<p>...</p>
</body>
</html>
Yeah, it is a mess
I’m proud that I was able to get this work way back in the day. I had a goal and I acheived it, regardless of how it was actually implemented. To me, that is what software development is about, as well as adapting and improving over time much like this code needs to do. I’ve updated the code a little just to make it work with my new site.